Introduction to Max Heap
A max heap is a binary tree-based data structure in which the parent node is always greater than or equal to its child nodes. This ensures that the maximum element is always at the root of the heap. python max heap are commonly used in priority queues, heap sort, and other algorithmic applications.
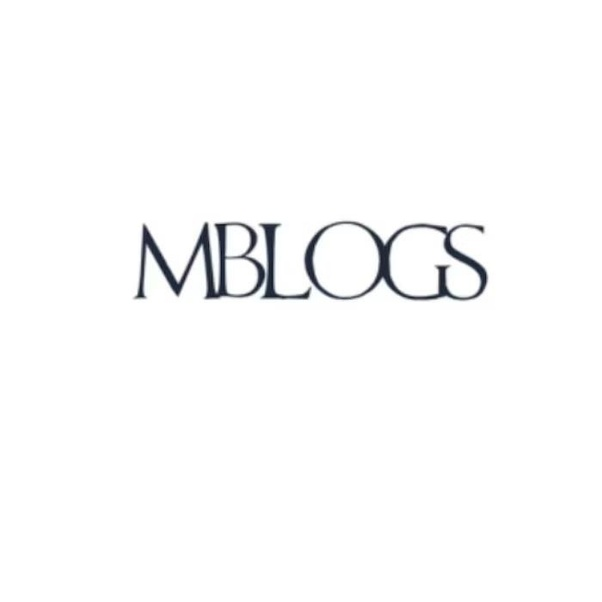
How Max Heap Works
A max heap follows a complete binary tree structure, meaning all levels of the tree are fully filled except possibly the last level, which is filled from left to right. The largest element is always at the root, and inserting or deleting elements maintains this property.
Implementing Max Heap in Python
Python’s heapq
module provides a min heap by default. However, a max heap can be implemented by negating the values before inserting them into the heap. This way, the smallest negative number (which represents the largest original number) remains at the root.
Operations in Max Heap
Common operations in a max heap include insertion, deletion, heapify, and extracting the maximum element. Each of these operations ensures that the heap property is maintained, preventing the structure from becoming an unordered binary tree.
Time Complexity of Max Heap
Max heap operations are efficient, with insertion and deletion both taking O(log n) time due to the need for reordering the elements to maintain the heap property. Accessing the maximum element is an O(1) operation since it is always at the root.
Use Cases of Max Heap
Max heaps are widely used in algorithms like heap sort, implementing priority queues, and solving problems that require frequent access to the largest element, such as scheduling systems and real-time processing.
Conclusion: Why Use a Max Heap?
Max heaps offer an efficient way to manage large datasets where priority-based access to the highest value is required. By utilizing Python’s heapq
with negation or implementing a custom heap, developers can take advantage of its performance benefits in various applications.